+{{#zone "bottomJs"}}
+ {{js "js/platform-configuration.js"}}
+{{/zone}}
diff --git a/components/device-mgt/org.wso2.carbon.device.mgt.ui/src/main/resources/jaggeryapps/devicemgt/app/units/cdmf.unit.platform.configuration/public/js/platform-configuration.js b/components/device-mgt/org.wso2.carbon.device.mgt.ui/src/main/resources/jaggeryapps/devicemgt/app/units/cdmf.unit.platform.configuration/public/js/platform-configuration.js
new file mode 100644
index 0000000000..f2704873a4
--- /dev/null
+++ b/components/device-mgt/org.wso2.carbon.device.mgt.ui/src/main/resources/jaggeryapps/devicemgt/app/units/cdmf.unit.platform.configuration/public/js/platform-configuration.js
@@ -0,0 +1,120 @@
+/*
+ * Copyright (c) 2016, WSO2 Inc. (http://www.wso2.org) All Rights Reserved.
+ *
+ * WSO2 Inc. licenses this file to you under the Apache License,
+ * Version 2.0 (the "License"); you may not use this file except
+ * in compliance with the License.
+ * You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing,
+ * software distributed under the License is distributed on an
+ * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
+ * KIND, either express or implied. See the License for the
+ * specific language governing permissions and limitations
+ * under the License.
+ */
+
+$(document).ready(function () {
+
+ var configParams = {
+ "NOTIFIER_TYPE": "notifierType",
+ "NOTIFIER_FREQUENCY": "notifierFrequency"
+ };
+
+ var responseCodes = {
+ "CREATED": "Created",
+ "SUCCESS": "201",
+ "INTERNAL_SERVER_ERROR": "Internal Server Error"
+ };
+
+ /**
+ * Checks if provided input is valid against RegEx input.
+ *
+ * @param regExp Regular expression
+ * @param inputString Input string to check
+ * @returns {boolean} Returns true if input matches RegEx
+ */
+ function isPositiveInteger(str) {
+ return /^\+?(0|[1-9]\d*)$/.test(str);
+ }
+
+ invokerUtil.get(
+ "/devicemgt_admin/configuration",
+ function (data) {
+ data = JSON.parse(data);
+ if (data && data.configuration) {
+ for (var i = 0; i < data.configuration.length; i++) {
+ var config = data.configuration[i];
+ if (config.name == configParams["NOTIFIER_FREQUENCY"]) {
+ $("input#monitoring-config-frequency").val(config.value / 1000);
+ }
+ }
+ }
+ }, function (data) {
+ console.log(data);
+ });
+
+ /**
+ * Following click function would execute
+ * when a user clicks on "Save" button
+ * on General platform configuration page in WSO2 EMM Console.
+ */
+ $("button#save-general-btn").click(function () {
+ var notifierFrequency = $("input#monitoring-config-frequency").val();
+ var errorMsgWrapper = "#email-config-error-msg";
+ var errorMsg = "#email-config-error-msg span";
+
+ if (!notifierFrequency) {
+ $(errorMsg).text("Monitoring frequency is a required field. It cannot be empty.");
+ $(errorMsgWrapper).removeClass("hidden");
+ } else if (!isPositiveInteger(notifierFrequency)) {
+ $(errorMsg).text("Provided monitoring frequency is invalid. ");
+ $(errorMsgWrapper).removeClass("hidden");
+ } else {
+ var addConfigFormData = {};
+ var configList = new Array();
+
+ var monitorFrequency = {
+ "name": configParams["NOTIFIER_FREQUENCY"],
+ "value": String((notifierFrequency * 1000)),
+ "contentType": "text"
+ };
+
+ configList.push(monitorFrequency);
+ addConfigFormData.configuration = configList;
+
+ var addConfigAPI = "/devicemgt_admin/configuration";
+ invokerUtil.post(
+ addConfigAPI,
+ addConfigFormData,
+ function (data) {
+ data = JSON.parse(data);
+ if (data.statusCode == responseCodes["SUCCESS"]) {
+ $("#config-save-form").addClass("hidden");
+ $("#record-created-msg").removeClass("hidden");
+ } else if (data == 500) {
+ $(errorMsg).text("Exception occurred at backend.");
+ } else if (data == 403) {
+ $(errorMsg).text("Action was not permitted.");
+ } else {
+ $(errorMsg).text("An unexpected error occurred.");
+ }
+
+ $(errorMsgWrapper).removeClass("hidden");
+ }, function (data) {
+ data = data.status;
+ if (data == 500) {
+ $(errorMsg).text("Exception occurred at backend.");
+ } else if (data == 403) {
+ $(errorMsg).text("Action was not permitted.");
+ } else {
+ $(errorMsg).text("An unexpected error occurred.");
+ }
+ $(errorMsgWrapper).removeClass("hidden");
+ }
+ );
+ }
+ });
+});
diff --git a/components/device-mgt/org.wso2.carbon.device.mgt.ui/src/main/resources/jaggeryapps/devicemgt/app/units/cdmf.unit.user.create/public/js/bottomJs.js b/components/device-mgt/org.wso2.carbon.device.mgt.ui/src/main/resources/jaggeryapps/devicemgt/app/units/cdmf.unit.user.create/public/js/bottomJs.js
index 32c7106125..489eee0bc2 100644
--- a/components/device-mgt/org.wso2.carbon.device.mgt.ui/src/main/resources/jaggeryapps/devicemgt/app/units/cdmf.unit.user.create/public/js/bottomJs.js
+++ b/components/device-mgt/org.wso2.carbon.device.mgt.ui/src/main/resources/jaggeryapps/devicemgt/app/units/cdmf.unit.user.create/public/js/bottomJs.js
@@ -254,7 +254,7 @@ $(document).ready(function () {
if (data.errorMessage) {
$(errorMsg).text("Selected user store prompted an error : " + data.errorMessage);
$(errorMsgWrapper).removeClass("hidden");
- } else if (data["status"] == 201) {
+ } else if (data["statusCode"] == 201) {
// Clearing user input fields.
$("input#username").val("");
$("input#firstname").val("");
@@ -265,17 +265,17 @@ $(document).ready(function () {
$("#user-create-form").addClass("hidden");
$("#user-created-msg").removeClass("hidden");
generateQRCode("#user-created-msg .qr-code");
- } else if (data["status"] == 409) {
+ } else if (data["statusCode"] == 409) {
$(errorMsg).text(data["messageFromServer"]);
$(errorMsgWrapper).removeClass("hidden");
- } else if (data["status"] == 500) {
+ } else if (data["statusCode"] == 500) {
$(errorMsg).text("An unexpected error occurred at backend server. Please try again later.");
$(errorMsgWrapper).removeClass("hidden");
}
}, function (data) {
- if (data["status"] == 409) {
+ if (data["statusCode"] == 409) {
$(errorMsg).text("User : " + username + " already exists. Pick another username.");
- } else if (data["status"] == 500) {
+ } else if (data["statusCode"] == 500) {
$(errorMsg).text("An unexpected error occurred at backend server. Please try again later.");
} else {
$(errorMsg).text(data.errorMessage);
diff --git a/components/device-mgt/org.wso2.carbon.device.mgt.ui/src/main/resources/jaggeryapps/devicemgt/jaggery.conf b/components/device-mgt/org.wso2.carbon.device.mgt.ui/src/main/resources/jaggeryapps/devicemgt/jaggery.conf
index 10170e9457..28ddcefb6c 100644
--- a/components/device-mgt/org.wso2.carbon.device.mgt.ui/src/main/resources/jaggeryapps/devicemgt/jaggery.conf
+++ b/components/device-mgt/org.wso2.carbon.device.mgt.ui/src/main/resources/jaggeryapps/devicemgt/jaggery.conf
@@ -11,10 +11,6 @@
"url": "/api/groups/*",
"path": "/api/group-api.jag"
},
- {
- "url": "/api/operations/*",
- "path": "/api/operation-api.jag"
- },
{
"url": "/api/policies/*",
"path": "/api/policy-api.jag"
diff --git a/components/policy-mgt/org.wso2.carbon.policy.mgt.common/pom.xml b/components/policy-mgt/org.wso2.carbon.policy.mgt.common/pom.xml
index cfa51b818b..597ea8239a 100644
--- a/components/policy-mgt/org.wso2.carbon.policy.mgt.common/pom.xml
+++ b/components/policy-mgt/org.wso2.carbon.policy.mgt.common/pom.xml
@@ -53,6 +53,8 @@
${carbon.device.mgt.version}
Policy Management Common Bundle
+ javax.xml.bind.annotation,
+ io.swagger.annotations.*;resolution:=optional,
org.wso2.carbon.device.mgt.common.*,
org.wso2.carbon.device.mgt.core.dto.*,
javax.xml.bind.*,
@@ -91,6 +93,11 @@
org.wso2.carbon.devicemgt
org.wso2.carbon.device.mgt.core
+
+ io.swagger
+ swagger-annotations
+ provided
+
diff --git a/components/policy-mgt/org.wso2.carbon.policy.mgt.common/src/main/java/org/wso2/carbon/policy/mgt/common/Policy.java b/components/policy-mgt/org.wso2.carbon.policy.mgt.common/src/main/java/org/wso2/carbon/policy/mgt/common/Policy.java
index 25c5f48cc4..4620adb72e 100644
--- a/components/policy-mgt/org.wso2.carbon.policy.mgt.common/src/main/java/org/wso2/carbon/policy/mgt/common/Policy.java
+++ b/components/policy-mgt/org.wso2.carbon.policy.mgt.common/src/main/java/org/wso2/carbon/policy/mgt/common/Policy.java
@@ -17,6 +17,8 @@
*/
package org.wso2.carbon.policy.mgt.common;
+import io.swagger.annotations.ApiModel;
+import io.swagger.annotations.ApiModelProperty;
import org.wso2.carbon.device.mgt.common.Device;
import javax.xml.bind.annotation.XmlElement;
@@ -29,24 +31,50 @@ import java.util.Map;
* This class will be the used to create policy object with relevant information for evaluating.
*/
@XmlRootElement
+@ApiModel(value = "Policy", description = "This class carries all information related to Policies")
public class Policy implements Comparable, Serializable {
private static final long serialVersionUID = 19981017L;
+ @ApiModelProperty(name = "id", value = "The policy ID", required = true)
private int id; // Identifier of the policy.
- private int priorityId; // Priority of the policies. This will be used only for simple evaluation.
+ @ApiModelProperty(name = "priorityId", value = "The priority order of the policy. 1 indicates the highest"
+ + " priority", required = true)
+ private int priorityId; // Priority of the policies. This will be used only for simple evaluation.
+ @ApiModelProperty(name = "profile", value = "Contains the details of the profile that is included in the "
+ + "policy", required = true)
private Profile profile; // Profile
+ @ApiModelProperty(name = "policyName", value = "The name of the policy", required = true)
private String policyName; // Name of the policy.
+ @ApiModelProperty(name = "generic", value = "If true, this should be applied to all related device",
+ required = true)
private boolean generic; // If true, this should be applied to all related device.
+ @ApiModelProperty(name = "roles", value = "The roles to whom the policy is applied on", required = true)
private List roles; // Roles which this policy should be applied.
+ @ApiModelProperty(name = "ownershipType", value = "The policy ownership type. It can be any of the "
+ + "following values:\n"
+ + "ANY - The policy will be applied on the BYOD and COPE device types\n"
+ + "BYOD (Bring Your Own Device) - The policy will only be applied on the BYOD device type\n"
+ + "COPE (Corporate-Owned, Personally-Enabled) - The policy will only be applied on the COPE "
+ + "device type\n", required = true)
private String ownershipType; // Ownership type (COPE, BYOD, CPE)
+ @ApiModelProperty(name = "devices", value = "Lists out the devices the policy is enforced on",
+ required = true)
private List devices; // Individual devices this policy should be applied
+ @ApiModelProperty(name = "users", value = "Lists out the users on whose devices the policy is enforced",
+ required = true)
private List users;
+ @ApiModelProperty(name = "active", value = "If the value is true it indicates that the policy is active. "
+ + "If the value is false it indicates that the policy is inactive", required = true)
private boolean active;
+ @ApiModelProperty(name = "updated", value = "If you have made changes to the policy but have not applied"
+ + " these changes to the devices that are registered with EMM, then the value is defined as true."
+ + " But if you have already applied any changes made to the policy then the value is defined as"
+ + " false.", required = true)
private boolean updated;
+ @ApiModelProperty(name = "description", value = "Gives a description on the policy", required = true)
private String description;
-
/* Compliance data*/
private String compliance;
diff --git a/components/policy-mgt/org.wso2.carbon.policy.mgt.common/src/main/java/org/wso2/carbon/policy/mgt/common/ProfileFeature.java b/components/policy-mgt/org.wso2.carbon.policy.mgt.common/src/main/java/org/wso2/carbon/policy/mgt/common/ProfileFeature.java
index 00e3b65d96..5de755a35d 100644
--- a/components/policy-mgt/org.wso2.carbon.policy.mgt.common/src/main/java/org/wso2/carbon/policy/mgt/common/ProfileFeature.java
+++ b/components/policy-mgt/org.wso2.carbon.policy.mgt.common/src/main/java/org/wso2/carbon/policy/mgt/common/ProfileFeature.java
@@ -18,16 +18,29 @@
package org.wso2.carbon.policy.mgt.common;
+import io.swagger.annotations.ApiModel;
+import io.swagger.annotations.ApiModelProperty;
+
import java.io.Serializable;
+@ApiModel(value = "ProfileFeature", description = "This class carries all information related to profile "
+ + "features")
public class ProfileFeature implements Serializable {
private static final long serialVersionUID = 19981018L;
+ @ApiModelProperty(name = "id", value = "Define the ID", required = true)
private int id;
+ @ApiModelProperty(name = "featureCode", value = "Provide the code that defines the policy you wish to add",
+ required = true)
private String featureCode;
+ @ApiModelProperty(name = "profileId", value = "Define the ID of the profile", required = true)
private int profileId;
+ @ApiModelProperty(name = "deviceTypeId", value = "The ID used to define the type of the device platform",
+ required = true)
private int deviceTypeId;
+ @ApiModelProperty(name = "content", value = "The list of parameters that define the policy",
+ required = true)
private Object content;
public int getId() {
diff --git a/features/device-mgt/org.wso2.carbon.device.mgt.server.feature/src/main/resources/email/templates/user-enrollment.vm b/features/device-mgt/org.wso2.carbon.device.mgt.server.feature/src/main/resources/email/templates/user-enrollment.vm
deleted file mode 100644
index 25b3b26a0c..0000000000
--- a/features/device-mgt/org.wso2.carbon.device.mgt.server.feature/src/main/resources/email/templates/user-enrollment.vm
+++ /dev/null
@@ -1,72 +0,0 @@
-#*
- Copyright (c) 2016, WSO2 Inc. (http://www.wso2.org) All Rights Reserved.
-
- WSO2 Inc. licenses this file to you under the Apache License,
- Version 2.0 (the "License"); you may not use this file except
- in compliance with the License.
- you may obtain a copy of the License at
-
- http://www.apache.org/licenses/LICENSE-2.0
-
- Unless required by applicable law or agreed to in writing,
- software distributed under the License is distributed on an
- "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
- KIND, either express or implied. See the License for the
- specific language governing permissions and limitations
- under the License.
-*#
-
- You have been invited to enroll your device in WSO2 EMM
-
-
-
- WSO2 Enterprise Mobility Manager
-
-
-
-
-
-
-
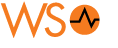
-
-
-
-
- Hi $first-name,
-
-
-
- You have been invited to enrol your device in WSO2 Enterprise Mobility Manager.
- Click here to download the WSO2 EMM client application to begin device
- enrolment.
-
-
- Should you need assistance, please contact your administrator.
-
-
-
- Regards,
-
-
-
- WSO2 EMM Administrator
-
-
-
-
-
-  |
-
-
-
-
-
-
-
- ]]>
-